C, Turtles
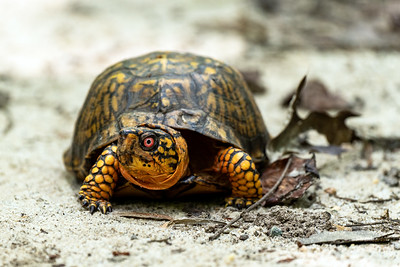
Mike Maguire
Getting started with programming turtle robots in C.
Monday...
I've randomly shuffled the cards to assign you partners:
- Zoey - Xu Dong
- Shoaib - Elise
- Mira - Abel
- Adrian - Karitas
- Marlon - Kevin
- Nate - Alejo
- Eric - Evan
Your assignment today:
Follow the instructions and read the reference material below:
- Install all the libraries needed by the turtle bot on your laptop (or on one of the lab computers)
- Download RobotTestMenu2015A.ino to your computer. Then send it to your robot.
- Try out the menu items in the RobotTestMenu and check that each one works for your robot.
- Write and install a new menu item to make your robot dance! : That is, move around in a distinctive way of your choosing for a few seconds.
- To 'hand in' your work, take a video of your robot moving and upload that and a copy of the new menu item you wrote.
After today, you should be ready to do the prelab for Lab 08. (And you may test your pre-lab code on a turtle bot, if you wish).
Libraries needed
Wire.h
andServo.h
come with Arduino and we've been using them so no action needed.Adafruit_RGBLCDShield.h
for the display shield. You have installed this for earlier labs. (See Lab 02 - prelab for installation instructions).physMenu.h
. You have installed this for earlier labs. (See Lab 02 - prelab for installation instructions).- New!
Adafruit_MotorShield.h
The turtle bots use an Adafruit board (shield) that can control all kinds of motors. You'll use it to control the wheels on the Turtle bots. There's a library to go with this new shield:
- Go to the Library Manager
- Search for adafruit motor
- We want the V2 Library
- Drop down the versions menu (it's probably marked "1.1.1") on the V2 Library entry. Choose version 1.0.11. This slightly older version provides all the functions we need, and will require 3 fewer additional libraries than the latest version.
- INSTALL the library!
Robot test program
Once you've installed the libraries above, you're ready to download the Robot test program RobotTestMenu2015A.ino, which you'll find in the SketchesMenus folder from our class resources link.
- Download RobotTestMenu2015A.ino,
- Open it in the Arduino library,
- Connect a serial cable to the Arduino board on your robot,
- Then download it to your robot,
- And try it out!
Turtle Robot coding
This is reference material for all the coding you'll do in Lab 08. Today, we're only dealing with movement. But come back here for the pre-lab, and during Lab 08 to find info about reading and moving (with a servo) the rangefinder.
Global variable declarations
In the program preamble these lines must appear:Adafruit_MotorShield AFMS; //creates Motor Shield object Adafruit_DCMotor *leftWheel = AFMS.getMotor(1); // Left Wheel DC Motor on port 1 Adafruit_DCMotor *rightWheel = AFMS.getMotor(2); // Right Wheel DC Motor on port 2 Servo rangerServo; // creates a servo object called rangerServo
- What's that
*
?? C allows you to declare both variables as well as variables which contain the location in memory of some other variable. This latter kind of variable is called a pointer. This intro to pointers has examples.
Yeah, $C$ is very powerful, but also confusing on first contact.
We'll approach pointers with a "cookbook" mentality for now: We can use a recipe (code example) to do things with pointers without worrying too much about the details of how they really work at this point.
In setup()
... you need to have these lines to initialize things:
m.lcd.setBacklight(0x1); // Turns on the backlight. pinMode(3, OUTPUT); // Rangefinder trigger pinMode(4, INPUT); // Rangefinder response AFMS.begin(); // Initialize the motor shield rangerServo.attach(2); // Associates rangerServo with pin 2
To use the servo to aim the rangefinder:
rangerServo.write(angle); // angle for straight=90 right=0 left=180 delay( x ); // x=100 or 200 for short moves, more for longer moves
To read the rangefinder, John has written a function:
// returns the range in cm int getRange() { int rangecm; digitalWrite(3, HIGH); delayMicroseconds(20); digitalWrite(3,LOW); rangecm = pulseIn(4, HIGH); rangecm = rangecm / 58; return rangecm; }
In your program you can call it like this:
range = getRange();
To move the motors, you command each wheel separately. To go forward:
leftWheel->run(FORWARD); rightWheel->run(FORWARD);
To go backward::
leftWheel->run(BACKWARD); rightWheel->run(BACKWARD);
To rotate to the right:
leftWheel->run(FORWARD); rightWheel->run(BACKWARD);
To rotate to the left:
leftWheel->run(BACKWARD); rightWheel->run(FORWARD);
This all happens at whatever is the current motor speed. To change it:
leftWheel->setSpeed(wheelSpeed); // wheelSpeed=100 is medium, 255 is max rightWheel->setSpeed(wheelSpeed);if (! $homepage){ $stylesheet="/~paulmr/class/comments.css"; if (file_exists("/home/httpd/html/cment/comments.h")){ include "/home/httpd/html/cment/comments.h"; } } ?>